from htmd.ui import *
from moleculekit.config import config
config(viewer='webgl')
2024-06-11 15:54:19,768 - numexpr.utils - INFO - Note: NumExpr detected 20 cores but "NUMEXPR_MAX_THREADS" not set, so enforcing safe limit of 8.
2024-06-11 15:54:19,769 - numexpr.utils - INFO - NumExpr defaulting to 8 threads.
2024-06-11 15:54:19,878 - rdkit - INFO - Enabling RDKit 2022.09.1 jupyter extensions
Please cite HTMD: Doerr et al.(2016)JCTC,12,1845. https://dx.doi.org/10.1021/acs.jctc.6b00049
HTMD Documentation at: https://software.acellera.com/htmd/
You are on the latest HTMD version (2.3.28+2.g8caf4970a).
Building Barnase - Barstar for protein-protein interactions#
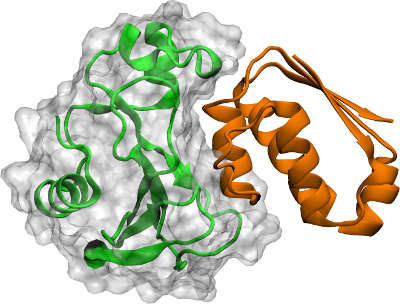
barnasebarstar#
Download the two proteins and view them#
You can look at their PDB information here and find out their PDB IDs. Then download them using those IDs.
You will need to create Molecule objects. Check the documentation on the Molecule class.
barnase = Molecule('2f4y')
barstar = Molecule('2hxx')
Filter the structures to keep only one chain of each#
Pick only one chain of each structure. This will keep the crystal waters corresponding to the chosen chain.
barnase.filter('chain A')
barstar.filter('chain A')
2024-06-11 15:54:26,540 - moleculekit.molecule - INFO - Removed 1919 atoms. 964 atoms remaining in the molecule.
2024-06-11 15:54:26,549 - moleculekit.molecule - INFO - Removed 743 atoms. 765 atoms remaining in the molecule.
array([ 719, 720, 721, 722, 723, 724, 725, 726, 727, 728, 729,
730, 731, 732, 733, 734, 735, 736, 737, 738, 739, 740,
741, 742, 743, 744, 745, 746, 747, 748, 749, 750, 751,
752, 753, 754, 755, 756, 757, 758, 759, 760, 761, 762,
763, 764, 765, 766, 767, 768, 769, 770, 771, 772, 773,
774, 775, 776, 777, 778, 779, 780, 781, 782, 783, 784,
785, 786, 787, 788, 789, 790, 791, 792, 793, 794, 795,
796, 797, 798, 799, 800, 801, 802, 803, 804, 805, 806,
807, 808, 809, 810, 811, 812, 813, 814, 815, 816, 817,
818, 819, 820, 821, 822, 823, 824, 825, 826, 827, 828,
829, 830, 831, 832, 833, 834, 835, 836, 837, 838, 839,
840, 841, 842, 843, 844, 845, 846, 847, 848, 849, 850,
851, 852, 853, 854, 855, 856, 857, 858, 859, 860, 861,
862, 863, 864, 865, 866, 867, 868, 869, 870, 871, 872,
873, 874, 875, 876, 877, 878, 879, 880, 881, 882, 883,
884, 885, 886, 887, 888, 889, 890, 891, 892, 893, 894,
895, 896, 897, 898, 899, 900, 901, 902, 903, 904, 905,
906, 907, 908, 909, 910, 911, 912, 913, 914, 915, 916,
917, 918, 919, 920, 921, 922, 923, 924, 925, 926, 927,
928, 929, 930, 931, 932, 933, 934, 935, 936, 937, 938,
939, 940, 941, 942, 943, 944, 945, 946, 947, 948, 949,
950, 951, 952, 953, 954, 955, 956, 957, 958, 959, 960,
961, 962, 963, 964, 965, 966, 967, 968, 969, 970, 971,
972, 973, 974, 975, 976, 977, 978, 979, 980, 981, 982,
983, 984, 985, 986, 987, 988, 989, 990, 991, 992, 993,
994, 995, 996, 997, 998, 999, 1000, 1001, 1002, 1003, 1004,
1005, 1006, 1007, 1008, 1009, 1010, 1011, 1012, 1013, 1014, 1015,
1016, 1017, 1018, 1019, 1020, 1021, 1022, 1023, 1024, 1025, 1026,
1027, 1028, 1029, 1030, 1031, 1032, 1033, 1034, 1035, 1036, 1037,
1038, 1039, 1040, 1041, 1042, 1043, 1044, 1045, 1046, 1047, 1048,
1049, 1050, 1051, 1052, 1053, 1054, 1055, 1056, 1057, 1058, 1059,
1060, 1061, 1062, 1063, 1064, 1065, 1066, 1067, 1068, 1069, 1070,
1071, 1072, 1073, 1074, 1075, 1076, 1077, 1078, 1079, 1080, 1081,
1082, 1083, 1084, 1085, 1086, 1087, 1088, 1089, 1090, 1091, 1092,
1093, 1094, 1095, 1096, 1097, 1098, 1099, 1100, 1101, 1102, 1103,
1104, 1105, 1106, 1107, 1108, 1109, 1110, 1111, 1112, 1113, 1114,
1115, 1116, 1117, 1118, 1119, 1120, 1121, 1122, 1123, 1124, 1125,
1126, 1127, 1128, 1129, 1130, 1131, 1132, 1133, 1134, 1135, 1136,
1137, 1138, 1139, 1140, 1141, 1142, 1143, 1144, 1145, 1146, 1147,
1148, 1149, 1150, 1151, 1152, 1153, 1154, 1155, 1156, 1157, 1158,
1159, 1160, 1161, 1162, 1163, 1164, 1165, 1166, 1167, 1168, 1169,
1170, 1171, 1172, 1173, 1174, 1175, 1176, 1177, 1178, 1179, 1180,
1181, 1182, 1183, 1184, 1185, 1186, 1187, 1188, 1189, 1190, 1191,
1192, 1193, 1194, 1195, 1196, 1197, 1198, 1199, 1200, 1201, 1202,
1203, 1204, 1205, 1206, 1207, 1208, 1209, 1210, 1211, 1212, 1213,
1214, 1215, 1216, 1217, 1218, 1219, 1220, 1221, 1222, 1223, 1224,
1225, 1226, 1227, 1228, 1229, 1230, 1231, 1232, 1233, 1234, 1235,
1236, 1237, 1238, 1239, 1240, 1241, 1242, 1243, 1244, 1245, 1246,
1247, 1248, 1249, 1250, 1251, 1252, 1253, 1254, 1255, 1256, 1257,
1258, 1259, 1260, 1261, 1262, 1263, 1264, 1265, 1266, 1267, 1268,
1269, 1270, 1271, 1272, 1273, 1274, 1275, 1276, 1277, 1278, 1279,
1280, 1281, 1282, 1283, 1284, 1285, 1286, 1287, 1288, 1289, 1290,
1291, 1292, 1293, 1294, 1295, 1296, 1297, 1298, 1299, 1300, 1301,
1302, 1303, 1304, 1305, 1306, 1307, 1308, 1309, 1310, 1311, 1312,
1313, 1314, 1315, 1316, 1317, 1318, 1319, 1320, 1321, 1322, 1323,
1324, 1325, 1326, 1327, 1328, 1329, 1330, 1331, 1332, 1333, 1334,
1335, 1336, 1337, 1338, 1339, 1340, 1341, 1342, 1343, 1344, 1345,
1346, 1347, 1348, 1349, 1350, 1351, 1352, 1353, 1354, 1355, 1356,
1357, 1358, 1359, 1360, 1361, 1362, 1363, 1364, 1365, 1366, 1367,
1368, 1369, 1370, 1371, 1372, 1373, 1374, 1375, 1376, 1377, 1378,
1379, 1380, 1381, 1382, 1383, 1384, 1385, 1386, 1387, 1388, 1389,
1390, 1391, 1392, 1393, 1394, 1395, 1396, 1397, 1398, 1399, 1400,
1401, 1402, 1403, 1404, 1405, 1406, 1407, 1408, 1409, 1410, 1411,
1412, 1413, 1414, 1415, 1416, 1417, 1418, 1419, 1420, 1421, 1422,
1423, 1424, 1425, 1426, 1427, 1428, 1429, 1430, 1431, 1432, 1433,
1434, 1435, 1436, 1437, 1484, 1485, 1486, 1487, 1488, 1489, 1490,
1491, 1492, 1493, 1494, 1495, 1496, 1497, 1498, 1499, 1500, 1501,
1502, 1503, 1504, 1505, 1506, 1507], dtype=int32)
Visualize the filtered structures#
from ipywidgets.widgets import Box; w = []
w.append(barnase.view())
w.append(barstar.view())
Box(children=(w[0],w[1]))
Box(children=(NGLWidget(), NGLWidget()))
Mutate modified residues#
Barstar has a modified residue for which we lack the parametrization (check under “Small Molecules” on PDB). Mutate the modified Tryptophan in Barstar (resname 4IN) to a normal Tryptophan (TRP):
barstar.mutateResidue('resname 4IN', 'TRP')
Assignments and renaming#
Assign a different chain (A, B) and segment to each protein (BRN, STR):
barnase.set('chain', 'A', 'protein')
barstar.set('chain', 'B', 'protein')
barnase.set('segid', 'BRN')
barstar.set('segid', 'STR')
Since the crystal waters were kept, assign them to segid W1 and W2 (assigning waters of both molecules to the same segid can cause problems as they will have same resids)
barnase.set('segid', 'W1', 'water')
barstar.set('segid', 'W2', 'water')
Combine the proteins and center them#
Create a new molecule which will contain both other molecules.
mol = Molecule()
mol.append(barnase)
mol.append(barstar)
Now center the new combined molecule on the origin.
mol.center()
Solvate the combined system#
Find the maximum distance of the atoms from the center:
from moleculekit.util import maxDistance
D = maxDistance(mol); print(D)
41.728752
Add 5 Å to this distance, to add some extra space in the box, and then solvate (no need to add a salt concentration):
D += 5
smol = solvate(mol, minmax=[[-D, -D, -D],[D, D, D]])
2024-06-11 15:54:43,847 - htmd.builder.solvate - INFO - Using water pdb file at: /home/sdoerr/Work/htmd/htmd/share/solvate/wat.pdb
2024-06-11 15:54:44,154 - htmd.builder.solvate - INFO - Replicating 8 water segments, 2 by 2 by 2
Solvating: 100%|█████████████████████████████████████████████████████████████████████████████████████████████████████████████████████████| 8/8 [00:02<00:00, 3.74it/s]
2024-06-11 15:54:46,896 - htmd.builder.solvate - INFO - 24833 water molecules were added to the system.
Build the solvated system in AMBER#
molbuilt = amber.build(smol, outdir='./build/')
2024-06-11 15:54:59,039 - htmd.builder.amber - INFO - Detecting disulfide bonds.
2024-06-11 15:55:00,515 - htmd.builder.amber - INFO - Starting the build.
2024-06-11 15:55:05,251 - htmd.builder.amber - INFO - Finished building.
2024-06-11 15:55:06,228 - moleculekit.writers - WARNING - Field "resid" of PDB overflows. Your data will be truncated to 4 characters.
2024-06-11 15:55:11,444 - htmd.builder.builder - WARNING - Found cis peptide bond in 1 frames: [0] in the omega diheral "Angle of (TYR 158 CA ) (TYR 158 C ) (PRO 159 N ) (PRO 159 CA ) " with indexes [2452, 2469, 2471, 2481]
2024-06-11 15:55:11,930 - htmd.builder.ionize - INFO - Adding 0 anions + 4 cations for neutralizing and 0 ions for the given salt concentration 0 M.
2024-06-11 15:55:14,245 - htmd.builder.amber - INFO - Starting the build.
2024-06-11 15:55:19,291 - htmd.builder.amber - INFO - Finished building.
2024-06-11 15:55:20,267 - moleculekit.writers - WARNING - Field "resid" of PDB overflows. Your data will be truncated to 4 characters.
2024-06-11 15:55:25,428 - htmd.builder.builder - WARNING - Found cis peptide bond in 1 frames: [0] in the omega diheral "Angle of (TYR 158 CA ) (TYR 158 C ) (PRO 159 N ) (PRO 159 CA ) " with indexes [2452, 2469, 2471, 2481]
/home/sdoerr/miniforge3/envs/htmd/lib/python3.10/site-packages/Bio/pairwise2.py:278: BiopythonDeprecationWarning: Bio.pairwise2 has been deprecated, and we intend to remove it in a future release of Biopython. As an alternative, please consider using Bio.Align.PairwiseAligner as a replacement, and contact the Biopython developers if you still need the Bio.pairwise2 module.
warnings.warn(
2024-06-11 15:55:28,866 - moleculekit.tools.sequencestructuralalignment - INFO - Alignment #0 was done on 197 residues: 2-200
Visualize the built system#
Can take a while to load…
molbuilt.view(sel='protein',style='NewCartoon',
color='Secondary Structure', hold=True)
molbuilt.view(sel='water',style='lines')
2024-06-11 15:55:29,459 - moleculekit.writers - WARNING - Field "resid" of PDB overflows. Your data will be truncated to 4 characters.
NGLWidget()